DynamoDB TTLを試す
DynamoDBのTTL機能を使うと、項目に期限を設定して、自動削除することができます。
※削除は期限切れになってから48時間以内に実行されます。
import boto3
import time
import uuid
from datetime import datetime, timedelta
try:
client = boto3.client('dynamodb')
table_name = 'my_table'
# テーブル作成
result = client.create_table(
AttributeDefinitions = [
{
'AttributeName': 'id',
'AttributeType': 'S'
},
],
TableName = table_name,
KeySchema = [
{
'AttributeName': 'id',
'KeyType': 'HASH'
},
],
ProvisionedThroughput = {
'ReadCapacityUnits': 3,
'WriteCapacityUnits': 3
}
)
print('create_table')
# テーブルができるまでスリープ
time.sleep(10)
# TTL有効化
result = client.update_time_to_live(
TableName = table_name,
TimeToLiveSpecification={
'Enabled': True,
'AttributeName': 'ttl'
}
)
print('update_time_to_live')
except Exception as e:
print(e)
try:
# データ追加
id = str(uuid.uuid4())
datetime_now = datetime.now()
ttl = datetime_now + timedelta(minutes=1) # 1分後
result = client.put_item(
TableName = table_name,
Item = {
'id': {'S': id},
'created_at': {'S': datetime_now.strftime("%Y/%m/%d %H:%M:%S")},
'unixtime': {'N': str(int(datetime_now.timestamp()))},
'ttl': {'N': str(int(ttl.timestamp()))},
}
)
print('put_item')
except Exception as e:
print(e)
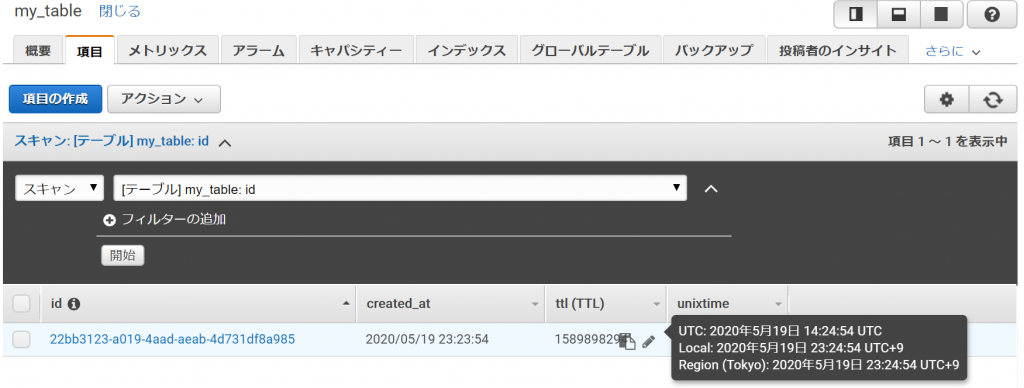
TTLに設定した属性はマウスオーバーすると読みやすい形式に変換して表示してくれます。
ディスカッション
コメント一覧
まだ、コメントがありません